Kotlin vs. Java: What To Choose for an Android App?
- What is Java?
- What is Kotlin?
- Kotlin versus Java: The programming languages comparison table
- Java vs. Kotlin: What programming language an Android developer recommends
- Developing an Android application from scratch
- Developing an Android application with pre-coded Java features
- Adding new functionality into an existing Android app
- Java vs Kotlin: What is the best language for Android app developers
To create Android apps, developers use either Java or Kotlin. When starting a new mobile project, you may wonder, “Should I build it on Java or use Kotlin instead?”
For me, as for the Android developer, the answer is obvious. For you, as for a person without a background in coding, the choice between those languages might not be so easy.
To draw you an overall picture, let me give you an example. When choosing between the old but gold Honda Accord and Tesla Model S, what would you select? I doubt you’ll choose the first option. Similarly, the answer for “Java vs. Kotlin-question” is clear to developers, but not to all C-levels and decision-makers.
I have written this article to give you a clue why most developers use Kotlin for new Android projects, and when Java code can still exist in your mobile application.
But first, let’s take a closer look at Java, Kotlin, and their main differences.
What is Java?
Java is an object-oriented programming language, named after an island in the Indian ocean.
The first Java release was 25 years ago, in 1995. Since then, Java has become widely used in the community of developers. And there are several reasons for its popularity:
- Simplicity (its code looks like C/C++)
- Wide range of applications (from mobile applications to database connectivity)
- Open-source libraries (logging, JSON parsing, unit testing, etc.)
VUE VS. ANGULAR VS. REACT COMPARISON
The following businesses are using Java in their products:
- Netflix uses Java for open-source tools and in the majority of the services within its architecture
- Uber leverages Java for developing Map Services, integrating external technologies, and analytics tools.
- Airbnb uses the language for their backend services in combination with the Dropwizard web service framework
- Google relies on Java for servers and the user interface
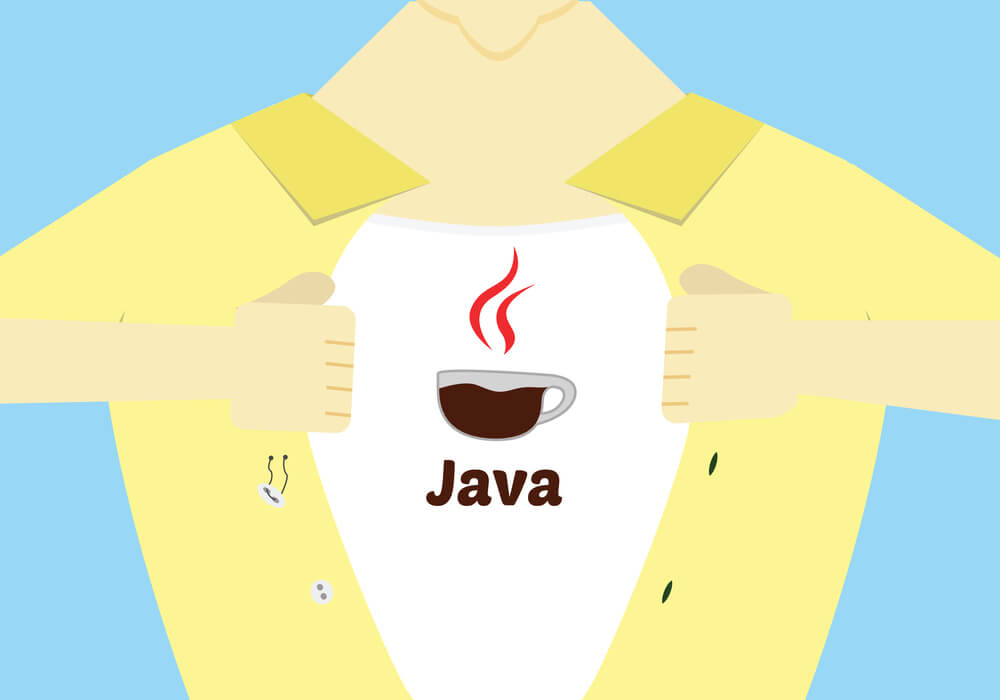
Java code example
Here is how Java Hello world code looks:
// Your First Program
class HelloWorld {
public static void main(String[] args) {
System.out.println(“Hello, World!”);
}
}
Now, let’s find out why mobile app developers like and don’t like Java at the same time.
What developers like about Java
I think that the Java language is not so bad for the following reasons:
Platform agnostic. I can use Java, not only for coding Android apps but also for web and cross-platform applications.
Established community. When I have an issue with code, I’ll find help in GitHub and Stack Overflow Java online communities.
Flexibility. I can build Java applications on Java Virtual Machine (JVM) and a browser window.
Code reusability. I can use code from one project in multiple apps that need it, which slightly reduces the development time.
What developers don’t like about Java
Developers almost hate Java. Really. Reddit’s thread “Why does everyone hate Java” includes 1700+ answers to this question. I find Java inconvenient for the following reasons:
Repetitive syntax. The code is drowned out in type declarations, temporary classes, and factories, which distracts me from fixing the actual problem.
Large code. When I write highly modular code with small cohesive methods, the code loses its readability and makes unit testing and code reuse extremely complicated.
Memory issues. The memory management happens through garbage collection, which slows down an app’s work.
Developers have used Java as an initial coding language for Android applications since the Android launch in 2008. Things changed in 2017, when Google, the official provider of Android Studio, announced Java is no longer a preferred language for developing apps. But what did Google recommend for creating Android applications instead of Java? Well, that’s Kotlin.
NODE JS VS. REACT COMPARISON: WHICH TO CHOOSE FOR YOUR JS PROJECT?
What is Kotlin?
Kotlin is a cross-platform statically typed programming language with open source code. The first release of Kotlin was in 2011 by JetBrains s.r.o., a Czech software development team.
In 2017, Kotlin became the official language for developing Android apps, announced at Google’s annual developer conference.
It is 100% interoperable with Java code. The JVM version of Kotlin’s standard library depends on Class Library written in Java. But, thanks to type inference, Kotlin syntax is more concise, which makes Kotlin the fourth most enjoyable language to use, says The annual Stack Overflow survey.
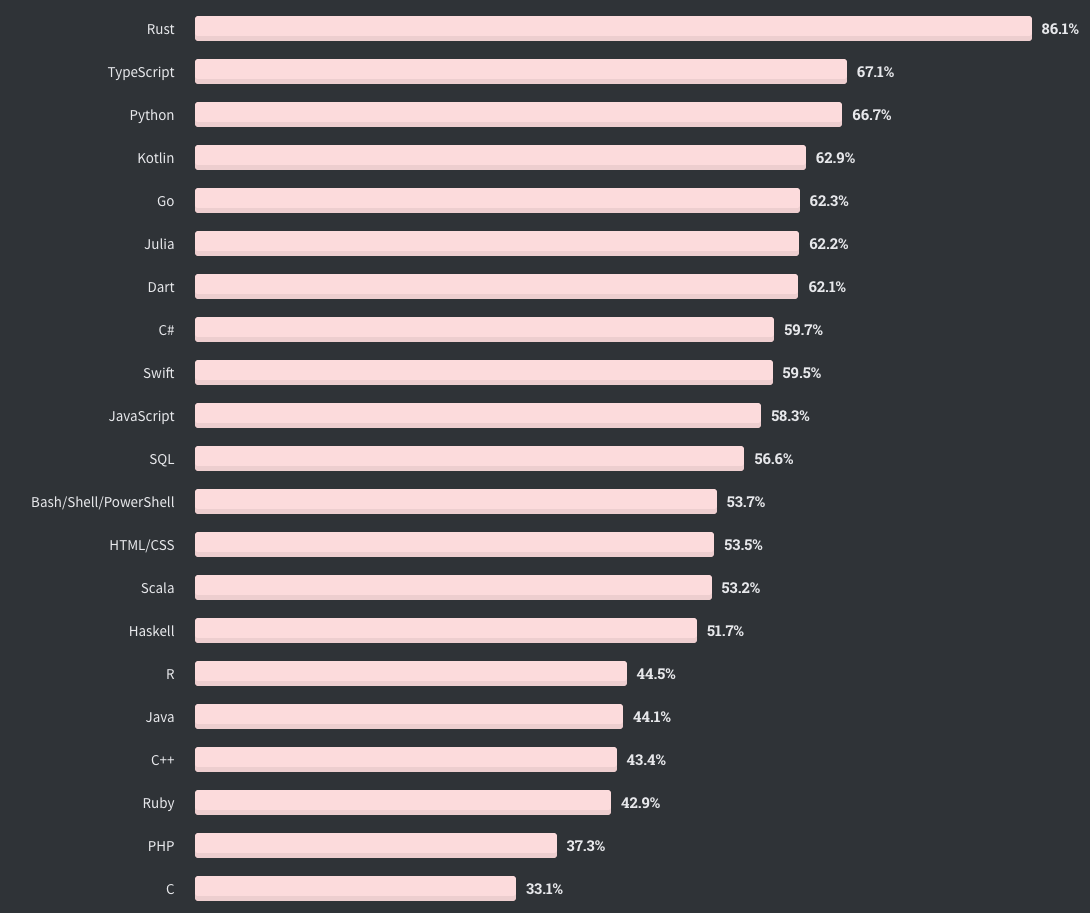
The following companies use Kotlin in their products already:
- Gradle applies Kotlin for writing build scripts
- Atlassian made an Android version of Trello in Kotlin
- Uber leveraged Kotlin for Android passenger and driver apps
- Pinterest has a Kotlin-made Android mobile application
Kotlin code example
One example of the Hello world Kotlin program looks like this:
// Hello World Program
fun main(args : Array
println(“Hello, World!”)
}
What developers like about Kotlin
I find Kotlin the best language for Android app development due to:
Less code. When I write code, I don’t need to create constructors for object initialization, classes to hold data, update, and retrieve values for declared fields. As a result, Kotlin code is 10%-20% shorter than Java code, which speeds up the development and deployment processes.
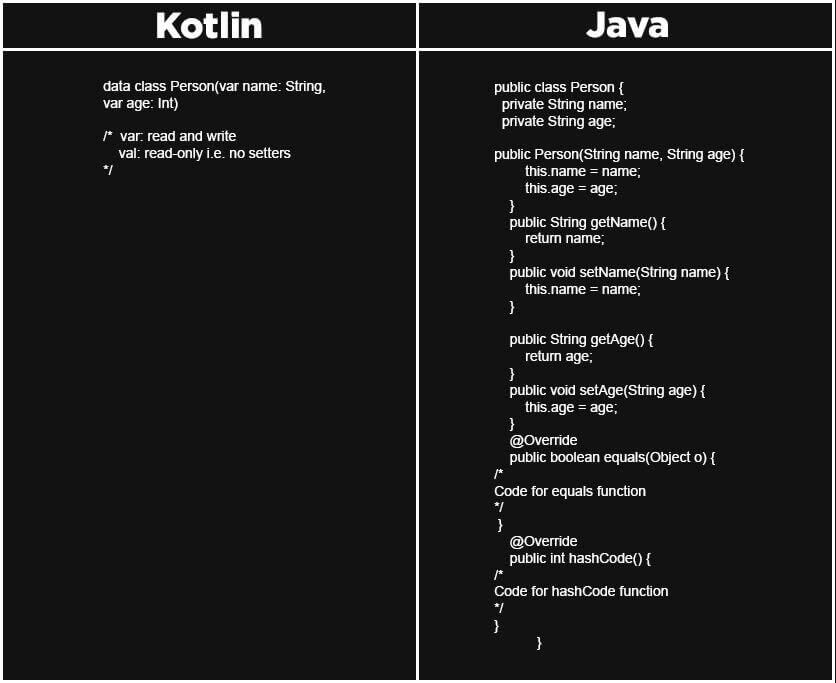
[Source]
Easy to maintain. Since I write less code, I make fewer mistakes and my code has fewer bugs. Less code also means lower app maintenance costs.
Simple in use. Kotlin eliminates Java-specific challenges, such as NullPointerException, and provides access to all frameworks and libraries written in Java for building clear and concise code.
Android Studio support. I use both Java and Kotlin code in the same project thanks to extended support and adaptation tools from Android Studio.
SWIFT VS REACT NATIVE: WHICH ONE IS BETTER TO CHOOSE WHEN DEVELOPING YOUR APP FOR IOS
What developers don’t like about Kotlin
Like everything in this world, Kotlin isn’t perfect. I find Kotlin adoption challenging for the following reasons:
Steep education path. Kotlin’s syntax is different from other programming languages, which complicates its adoption. It also means that hiring Kotlin developers requires more time.
Young community. In some cases, I can’t find answers in online community groups dedicated to Kotlin. But, I hope developers will solve this issue by contributing to Kotlin projects on GitHub and participating in issue discussions in StackOverflow.
Now, let’s compare how Java is different from Kotlin in terms of functionality.
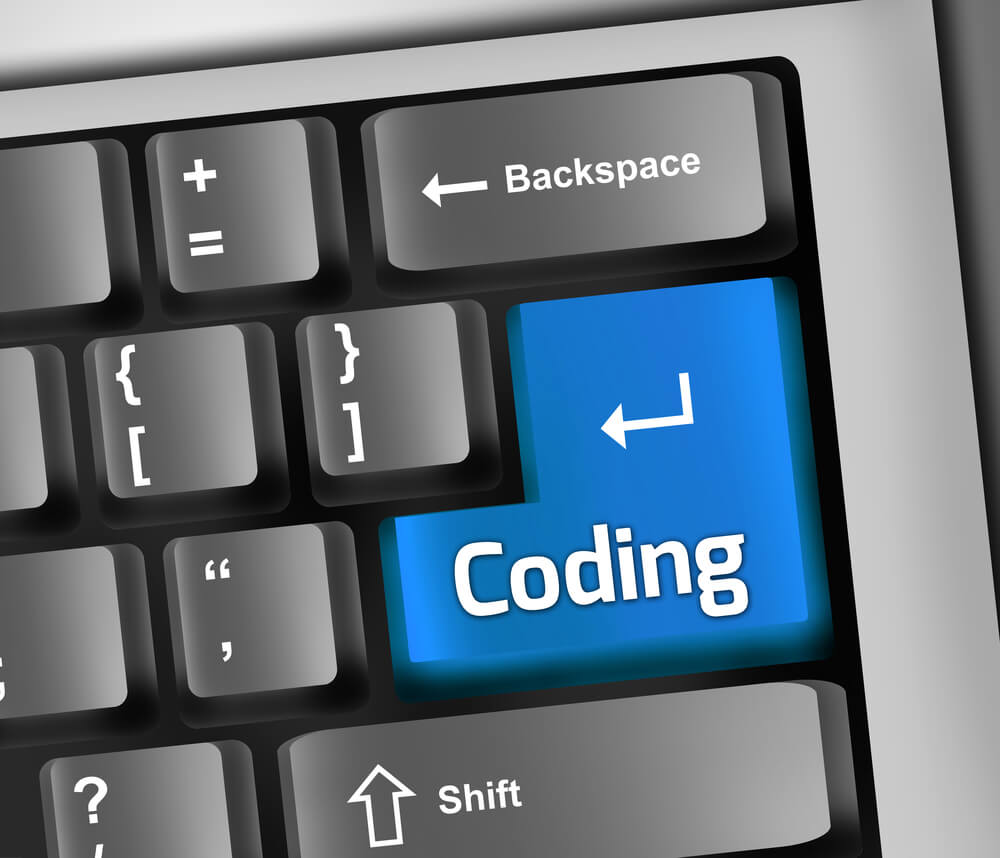
Kotlin vs. Java: the programming languages comparison table
In the table below, I included a detailed comparison of Java and Kotlin programming languages.
Differences | Java | Kotlin |
Null Safe | Includes a NullPointerExceptions that raises a null pointer exception when accessing an object reference with a null value. | Allows assigning null values to any variables/objects. |
Extension Functions | Requires creating a new class and inheriting the parent class, which means new functionality isn’t available. | Allows creating extended functions by prefixing the name of a class to the name of the new function. |
Coroutines Support | Blocks a corresponding thread during a long-running network I/0 and CPU Intensive operations. | Includes coroutines support that suspends execution without blocking threads while executing long-running intensive operations. |
Checked exceptions | Includes checked exceptions that declare and catch exceptions in code, which leads to a code with good error handling. | Doesn’t include checked exceptions. |
Data classes | Requires defining constructors, variables to store data, set methods, etc. | Declares a class with the keyword “data” in the class definition. Constructors, getter, and setter methods for different fields are created automatically. |
Smart casts | Requires checking the type of variables and casting according to an operation. | Includes smart casts for handling casting checks of immutable values and performing implicit casting. |
Type inference | Requires specifying each variables’ type explicitly. | Specifies the variable type explicitly based on the assignment it handles. |
Functional Programming | Provides functional programming support to only a subset of Java 8 features | Includes useful methods such as lambda, operator overloading, higher-order functions, etc. |
With that being said, developers find Kotlin a far more convenient programming language. Its syntax doesn’t require us to repeat the same code when writing a new one. Thus, the “Java vs. Kotlin” question hasn’t arisen in the developer community in recent years. But, mobile app development isn’t that simple in practice.
Before suggesting the relevant coding language for your project, I need to learn more about your business needs, expectations, and project goals. Moreover, there are even cases when I’ll recommend Java as the best option.
To understand why mobile app development isn’t black and white, check out several examples from my experience.
Related reading:
How to Outsource App Development Without Failing
How The APP Solutions team works: Cooperation Models and Development Phases
The Discovery Phase with The APP Solutions
Java vs. Kotlin: What programming language an Android developer recommends
You’ll need an Android developer in several cases – to build Android applications from the ground up or add more advanced functionality to an existing application. Depending on the business case, the answer to the question “Java vs Kotlin?” varies.
Developing an Android application from scratch
Before recommending the best programming language for new Android applications, the first thing I do is clarifying project deadlines, in-house dev team composition, and the budget.
Tight deadlines, limited budget, and a small development team without previous experience in Kotlin are signs that you need to start your project with Java. From a business perspective, Android app development will cost you less. Since most mobile developers know Java, they can start the project at once. At the same time, you save time and budget on hiring Kotlin-experienced programmers.
But, in the case of having enough time before the project launch, extra budget, and time for hiring additional developers, I recommend using Kotlin.
BACK-END TECH STACK FOR CUSTOM ELEARNING SOLUTIONS
Developing an Android application with pre-coded Java features
Some clients ask us to use existing Java algorithms, features, and plugins for their projects. At the same time, they want the code to be written on Kotlin but have no budget for re-building Java functionality.
In this case, I recommend starting your project with Kotlin, then integrating Java pre-coded app functionality. This approach works because Kotlin is compiled with Java bytecode and JVM (Java Virtual Machine).
To call existing Java code from Kotlin, developers use the following command:
import java.util.*
fun demo(source: List
val list = ArrayList
// ‘for’-loops work for Java collections:
for (item in source) {
list.add(item)
}
// Operator conventions work as well:
for (i in 0..source.size – 1) {
list[i] = source[i] // get and set are called
}
}
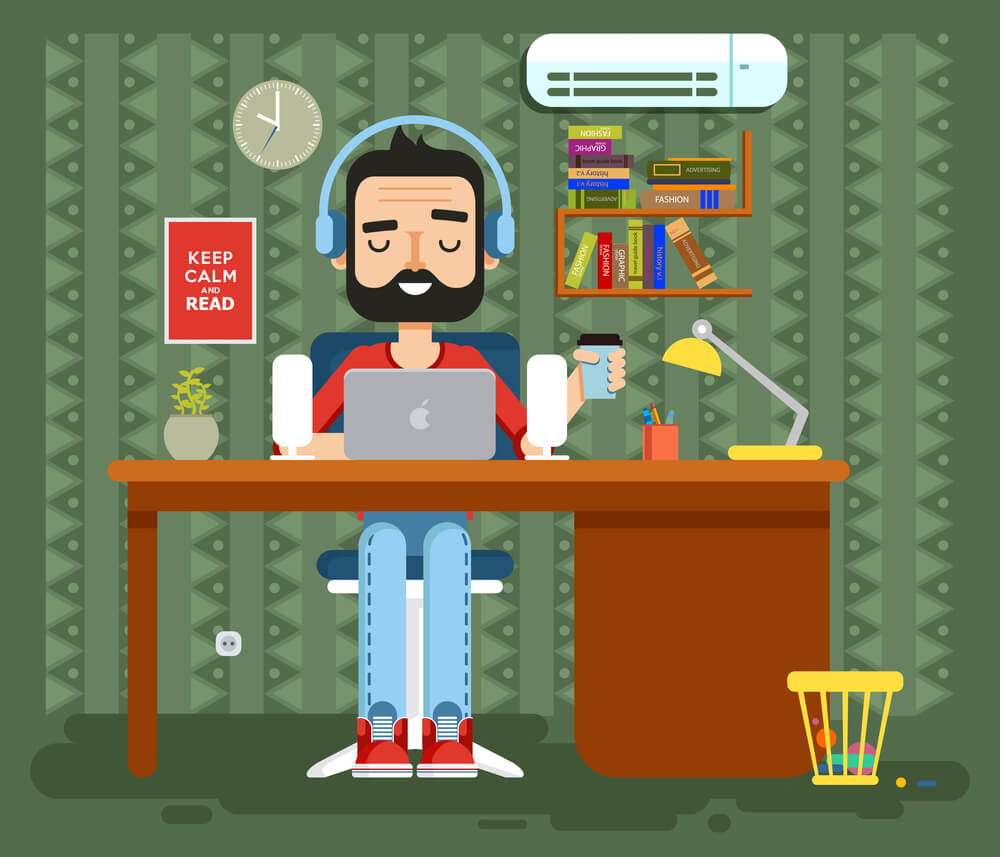
Adding new functionality into an existing Android app
If your application was launched before 2017, I’m 99.99% sure it is written in Java. Now, you might need to integrate new business functionality. So, you’ll face the dilemma – to hire Android developers to re-write the app with Kotlin language or integrate new functionality powered by Java? In both cases, the right answer is “no.”
As I mentioned earlier, Java and Kotlin codes work together. Thus, re-building your application with Kotlin to receive a 100% Kotlin-written app doesn’t make any sense. For adding new functionality, I suggest using Kotlin, since there are many examples of both programming languages co-existing in one mobile application.
One example is Passport Scan, the application we developed to streamline hotel check-in. The client challenged us with integrating a web application into an existing hotel management system, made with Java.
We began building the core features using Java. But soon, we switched to Kotlin for adding the new app’s functionality. Learn more about the project by reading the Passport Scan case study.
Download Free E-book with DevOps Checklist
Download NowKotlin vs Java: What is the best language for Android app developers
Kotlin programming language addresses Java’s weak points – in syntax and memory usage, as well as other imperfections. And Kotlin is doing it well. The growing community of Kotlin developers proves that when a developer learns Kotlin, one will never write on Java again.
WHAT TECH STACK TO CHOOSE FOR YOUR PROJECT
Kotlin-built projects take less time to code, require fewer bugs to fix, and cost less, compared with Java applications. In contrast, developers can write a new mobile application in the Java language as an exception to the rule.
If you have an idea for a Kotlin project and are looking for well-performing developers, drop us several lines. Let us know your idea, and we’ll figure out how we can be useful.